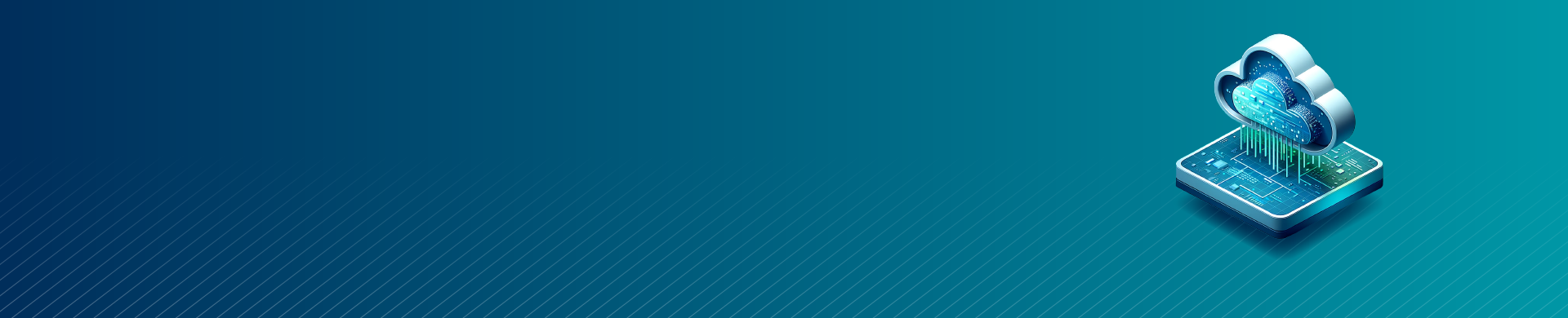
Data Obfuscation
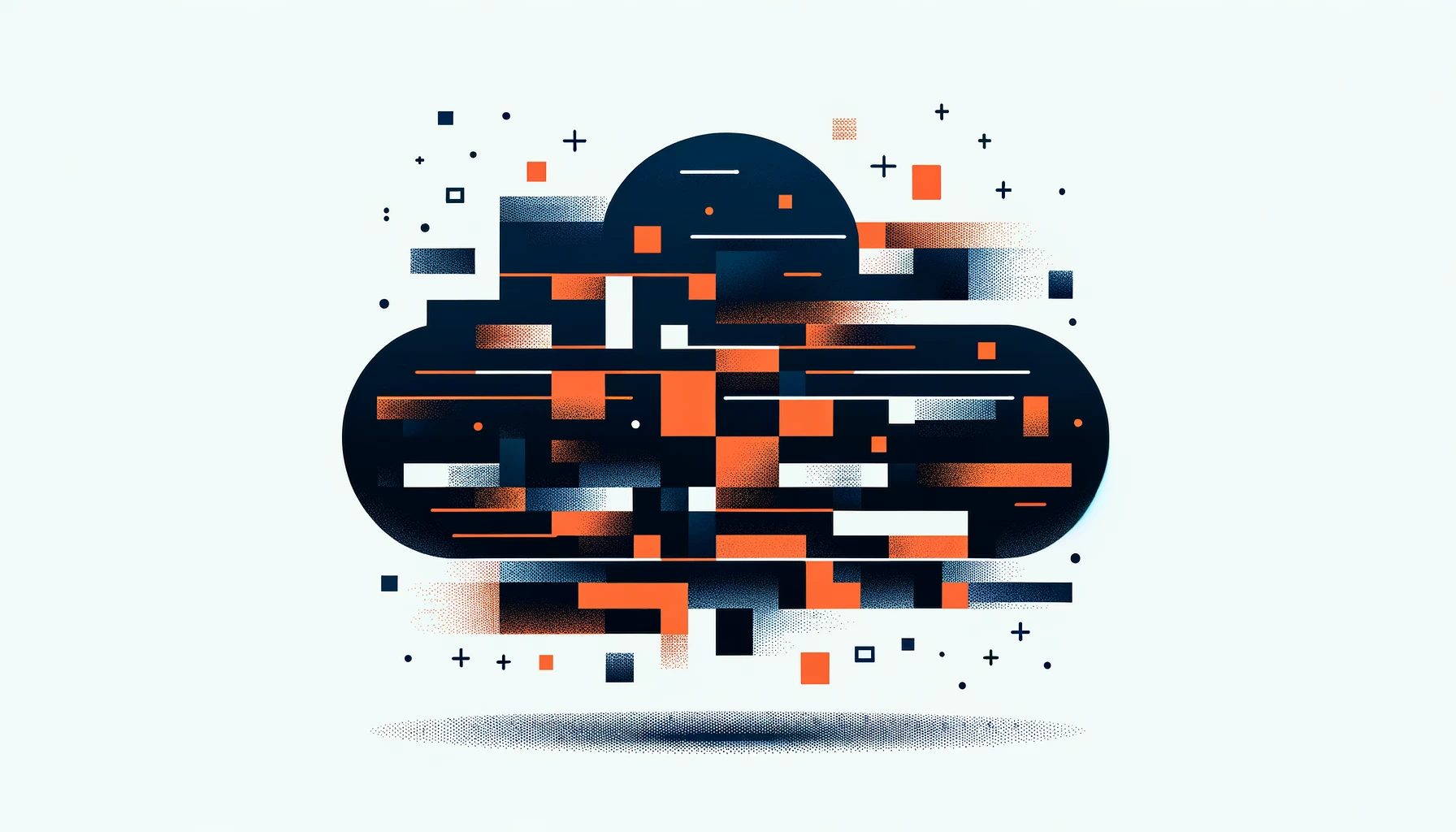
In today’s digital age, data security is of utmost importance. Cyber threats and data breaches are increasing. Organizations must take proactive measures to protect sensitive information stored in their databases. One effective technique for enhancing database security is data obfuscation, also known as data masking.
This article will explain the basics of data obfuscation. It will also discuss the benefits of data obfuscation. Additionally, it will show how to apply data obfuscation using command-line tools and the Python API for PostgreSQL.
What is Data Obfuscation?
Data obfuscation is when you hide important data in a database by replacing it with fake but believable information. The objective is to safeguard the original data from unauthorized access, while still ensuring its usability for testing, development, or analytics.
Companies can lower the risk of data leaks and follow privacy regulations like GDPR and HIPAA by keeping sensitive information secure. This can be achieved by implementing strong security measures and encryption protocols. By doing so, companies can protect their data from unauthorized access and potential breaches. This not only helps in maintaining compliance with regulations but also builds trust with customers and stakeholders.
Data Obfuscation vs. Data Masking
While people often use data obfuscation and data masking interchangeably, the two terms have a subtle difference. Data obfuscation is a broader concept that encompasses various techniques for obscuring sensitive data, including data masking.
Data masking is a method of data obfuscation that involves replacing sensitive data with realistic-looking fake values. The masked data has the same format and structure as the original data. This enables users to use it for testing and development purposes. Companies commonly use data masking to protect personally identifiable information (PII) such as names, addresses, and social security numbers.
Data obfuscation can involve more than just data masking. It can also include techniques like data encryption, data tokenization, and data shuffling. These techniques are used to protect sensitive data by altering it. This makes the data unreadable without the correct decryption key or mapping.
In summary, data masking is a specific technique within the broader category of data obfuscation. Data masking replaces sensitive data with realistic values, while data obfuscation uses methods like encryption, tokenization, and shuffling to protect data.
Types of Data Obfuscation
Various techniques conceal data. The method chosen depends on the type of data and its required level of security. Some common types include:
- Data Masking: This involves replacing sensitive data with fictitious but realistic-looking values. For example, you can use random names instead of real names. You can also use fake credit card numbers that are still valid.
- Data Encryption: Strong cryptographic algorithms encrypt sensitive data, making it unreadable without the appropriate decryption key. Even if someone accesses the database, nothing harmful can happen. That’s why we add this extra layer of protection.
- Tokenization replaces sensitive data with a unique, randomly generated token. You store the data safe in another system. And you use the token to find it when necessary. Businesses commonly use this method to protect payment card information.
- Data Shuffling is a technique that involves mixing up the values in a column randomly. This makes it difficult to identify specific individuals connected to the data. It is useful for preserving the statistical properties of the data while obscuring individual records.
Benefits of Data Obfuscation
Implementing data obfuscation offers several benefits for organizations:
- Enhanced Data Security: By obfuscating sensitive data, organizations can significantly reduce the risk of data breaches and unauthorized access. Even if an attacker gains access to the database, the obfuscated data will be of little value.
- Compliance with Regulations: Many industries have strict privacy regulations that require the protection of sensitive customer information. Data obfuscation helps organizations comply with these regulations by ensuring that sensitive data is not exposed.
- Improved Testing and Development: Obfuscated data allows developers and testers to work with realistic data without compromising the privacy of real individuals. This enables more effective testing and development processes while maintaining data security.
- Reduced Risk of Insider Threats: Data obfuscation limits the exposure of sensitive information to authorized personnel, reducing the risk of insider threats such as data theft or misuse.
Implementing Data Obfuscation with Command-Line Tools
One way to implement data obfuscation is by using command-line tools. Let’s consider an example using the PostgreSQL command-line client, psql.
Suppose we have a table named “customers” with columns “id”, “name”, “email”, and “phone”. To obfuscate the sensitive columns, we can use SQL commands to update the data.
-- Obfuscate customer names UPDATE customers SET name = 'Customer' || id; -- Obfuscate email addresses UPDATE customers SET email = 'customer' || id || '@example.com'; -- Obfuscate phone numbers UPDATE customers SET phone = '+1-555-' || LPAD(FLOOR(RANDOM() * 10000)::text, 4, '0');
The SQL statements above update the “name” column by replacing it with a generic “Customer” prefix followed by the unique “id”. The system updates the “email” column to a fictitious email address using the “id”. The “phone” column is made unclear by adding a random 4-digit number to a standard phone number prefix.
It’s important to note that before running these commands, you should create a backup of your database to ensure data integrity and the ability to restore the original data if needed.
Implementing Data Obfuscation with Python API for PostgreSQL
Another approach to data obfuscation is using the Python programming language and the psycopg2 library, which provides a PostgreSQL database adapter for Python. Here’s an example of how you can obfuscate data using Python:
import psycopg2 from faker import Faker # Establish a connection to the PostgreSQL database conn = psycopg2.connect( host="localhost", database="mydatabase", user="myuser", password="mypassword" ) # Create a cursor object to execute SQL queries cur = conn.cursor() # Initialize the Faker library for generating fictitious data fake = Faker() # Obfuscate customer names cur.execute("UPDATE customers SET name = %s || id", ('Customer',)) # Obfuscate email addresses cur.execute("UPDATE customers SET email = %s || id || %s", ('customer', '@example.com')) # Obfuscate phone numbers cur.execute("UPDATE customers SET phone = %s || LPAD(FLOOR(RANDOM() * 10000)::text, 4, '0')", ('+1-555-',)) # Commit the changes to the database conn.commit() # Close the cursor and database connection cur.close() conn.close()
In this example, we use the psycopg2 library to connect to a PostgreSQL database. We create a cursor object to execute SQL queries. The Faker library is used to generate fictitious data for obfuscation.
We execute SQL queries using the cursor object to update the “name”, “email”, and “phone” columns with obfuscated values. The changes are then committed to the database, and finally, the cursor and database connection are closed.
Detailed description of the queries
The line
cur.execute("UPDATE customers SET name = %s || id", ('Customer',))is an SQL query execution using the psycopg2 library in Python. Let’s break it down:
- cur.execute() is a method of the cursor object (cur) that executes an SQL query.
- The first argument to execute() is the SQL query string. In this case, it’s an UPDATE statement that modifies the “name” column of the “customers” table.
- The SQL query uses a parameterized query notation with %s as a placeholder. This is a best practice to prevent SQL injection attacks and improve performance.
- The || id part of the query concatenates the value of the “id” column with the value that will replace %s.
- The second argument to execute() is a tuple (‘Customer’,) that contains the value to be substituted for the %s placeholder in the SQL query. In this case, it’s the string ‘Customer’.
So, when this line is executed, it updates the “name” column of each row in the “customers” table by setting it to the concatenation of the string ‘Customer’ and the value of the “id” column for that row.
For example, if the “customers” table has the following data:
id | name | email | phone ---+-----------+------------------+---------- 1 | John | [email protected] | 123456789 2 | Alice | [email protected]| 987654321
After executing the SQL query, the “name” column will be updated as follows:
id | name | email | phone ---+-----------+------------------+---------- 1 | Customer1 | [email protected] | 123456789 2 | Customer2 | [email protected]| 987654321
The “name” column now contains obfuscated values that consist of the string ‘Customer’ followed by the respective “id” value for each row.
This is a simple example of data obfuscation where sensitive customer names are replaced with generic values while still maintaining a unique identifier (the “id” column) for each customer record.
Before running this Python script, make sure you have the necessary dependencies installed, such as psycopg2 and Faker, and that you have the appropriate database connection details.
Conclusion
Data obfuscation is a crucial technique for protecting sensitive information in databases and ensuring compliance with privacy regulations. By obscuring sensitive data with fictitious but realistic-looking values, organizations can significantly reduce the risk of data breaches and unauthorized access.
We explored the basics of data obfuscation, its benefits, and provided examples of how it can be implemented using command-line tools and the Python API for PostgreSQL. Whether you choose to use SQL commands or leverage the power of Python, data obfuscation is an essential tool in your database security arsenal.
DataSunrise
For exceptional and flexible data obfuscation solutions, consider DataSunrise. Dynamic data masking happens in real time as the user accesses the data. Static masking protects the data in the production database at rest. DataSunrise implements both data masking techniques.
DataSunrise provides a variety of tools for database security, including data obfuscation, audit rules, data masking, and compliance features. Contact the DataSunrise team and schedule an online demo. You can see how our solutions protect your sensitive data and secure your databases.