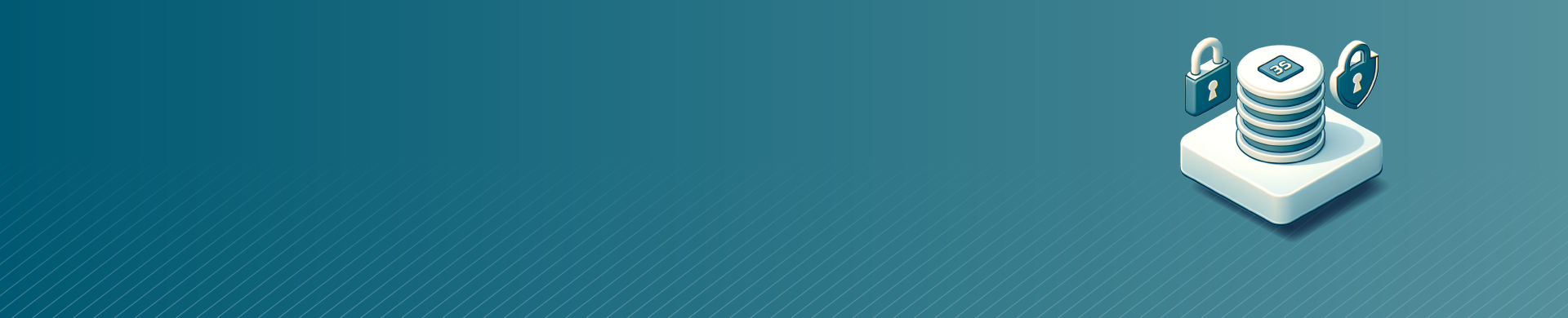
PostgreSQL Authentication
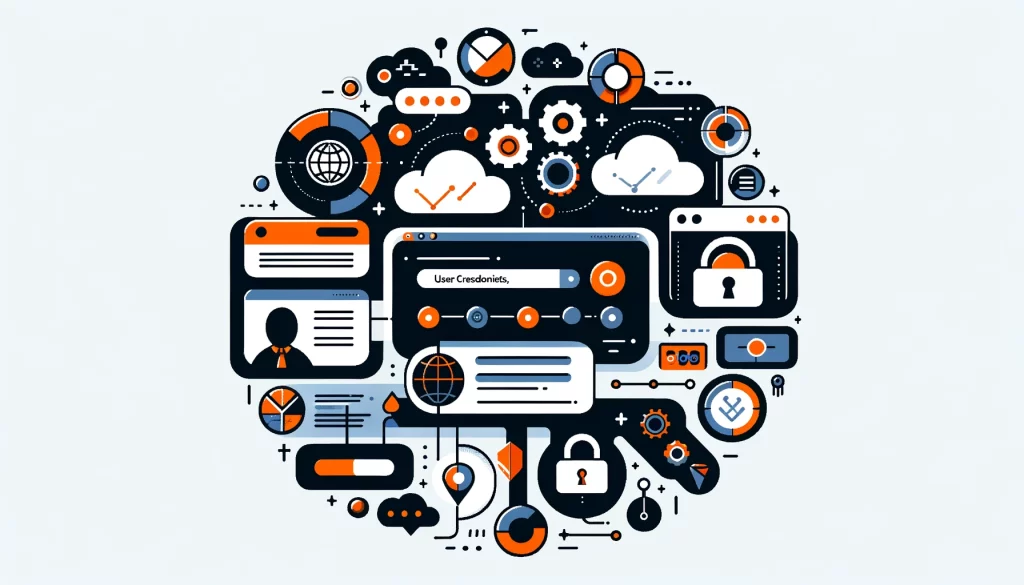
PostgreSQL is a powerful, open-source relational database management system known for its reliability, feature robustness, and performance. Organizations of all sizes use it for many different applications, from small web apps to large enterprise systems. A strong database can still be vulnerable if authentication is weak. PostgreSQL provides a comprehensive set of authentication methods to control access to your database and protect your sensitive data.
PostgreSQL Authentication Methods
The database supports a wide range of authentication methods, each with its own strengths and use cases. These methods are configured in the `pg_hba.conf` file, which determines how clients can connect to the database server. The `pg_hba.conf` file has rules that can be used to specify different authentication methods for databases, users, and connection types.
The main authentication methods in PostgreSQL are:
- Trust – This method allows anyone to connect without requiring a password. It’s only recommended for local development and testing environments where security is not a concern.
- Password – This method requires the user to provide a password to connect. By default, the password is transmitted in clear text, so it’s important to use SSL/TLS encryption to secure the connection.
- MD5 – Similar to the password method, but the password is hashed using the MD5 algorithm before being sent over the network. While more secure than clear text, MD5 is not considered a strong hashing algorithm by modern standards.
- SCRAM-SHA-256 – This is the most secure password-based authentication method in PostgreSQL. It uses the Salted Challenge Response Authentication Mechanism (SCRAM) with the SHA-256 hashing function to provide strong password security.
- Ident – This method uses the operating system’s ident service to get the client’s operating system user name, which is then used for authentication. It’s useful for systems where the database users mirror the operating system users.
- PostgreSQL uses methods like GSSAPI, SSPI, Kerberos, LDAP, RADIUS, Certificate, and PAM to connect with various authentication systems. These methods enable single sign-on and user management.
Each authentication method has its own configuration options and requirements. The `pg_hba.conf` file allows you to mix and match these methods based on your specific security and usability needs.
Implementing Password Authentication
For most applications, password-based authentication is the preferred choice and many PostgreSQL clients and connectors support it. It provides a good balance of security and usability. To enable password authentication, you need to:
- Create a user with a password:
CREATE USER myuser WITH PASSWORD 'secret123';
- Configure `pg_hba.conf` to use the `scram-sha-256` authentication method for this user:
# TYPE DATABASE USER ADDRESS METHOD host mydb myuser 0.0.0.0/0 scram-sha-256
This entry gives the user “myuser” permission to access the “mydb” database from any IP address using SCRAM-SHA-256 for authentication.
- Restart the PostgreSQL server for the changes to take effect.
Now, when “myuser” tries to connect to “mydb”, they will be prompted for their password. The server will verify the password using the SCRAM-SHA-256 method.
It’s crucial to use strong, unique passwords and to change them regularly. PostgreSQL provides the `ALTER USER` command to change a user’s password:
ALTER USER myuser WITH PASSWORD 'new_secret';
Avoid using easily guessable or common passwords, and consider implementing a password policy that requires a minimum length, complexity, and regular expiration.
Securing Connections with SSL/TLS
Password authentication over an unencrypted connection is vulnerable to interception and sniffing attacks. To stay safe, it’s recommended to use SSL/TLS to protect communication between the client and server.
To enable SSL in PostgreSQL:
- Generate a server certificate and key using a tool like OpenSSL. For testing, use a self-signed certificate. For production, use a certificate signed by a trusted Certificate Authority (CA).
- Place the certificate and key files in a secure location on the server, and set the appropriate file permissions.
- Edit the `postgresql.conf` file to enable SSL and point to the certificate and key files:
ssl = on ssl_cert_file = '/path/to/server.crt' ssl_key_file = '/path/to/server.key'
- Restart the PostgreSQL server for the changes to take effect.
- Configure `pg_hba.conf` to require SSL for the desired connections:
# TYPE DATABASE USER ADDRESS METHOD hostssl mydb myuser 0.0.0.0/0 scram-sha-256
The `hostssl` type is like `host`, but requires an SSL connection.
- Configure your PostgreSQL clients to use SSL. For example, with the `psql` command line client:
psql "dbname=mydb user=myuser sslmode=require"
The `sslmode` parameter can be `require` (always use SSL), `verify-ca` (like require, but also verify the server certificate), or `verify-full` (like verify-ca, but also verify that the server hostname matches the certificate).
When SSL is enabled, it encrypts all data transmitted between the client and server, including passwords and query results. This greatly reduces the risk of sensitive data being intercepted over the network.
Implementing Role-Based Access Control
In addition to authenticating users, PostgreSQL allows you to authorize their actions using role-based access control. With RBAC, you define roles that represent different user types or permission levels, and then grant privileges to those roles.
For example, you might have roles like “readonly”, “readwrite”, and “admin”:
CREATE ROLE readonly; GRANT SELECT ON ALL TABLES IN SCHEMA public TO readonly; CREATE ROLE readwrite; GRANT SELECT, INSERT, UPDATE, DELETE ON ALL TABLES IN SCHEMA public TO readwrite; CREATE ROLE admin; GRANT ALL PRIVILEGES ON DATABASE mydb TO admin;
You can then assign users to these roles:
CREATE USER user1 WITH PASSWORD 'secret1'; GRANT readonly TO user1; CREATE USER user2 WITH PASSWORD 'secret2'; GRANT readwrite TO user2; CREATE USER dba WITH PASSWORD 'secret_admin'; GRANT admin TO dba;
Users will inherit the privileges of the roles they are granted. This makes it easy to manage permissions for large numbers of users.
RBAC allows you to implement the principle of least privilege, where users are only granted the minimum permissions they need to perform their tasks. This reduces the risk of accidental or intentional misuse of privileges.
Advanced Authentication Techniques
Password authentication is usually enough for most applications, but sometimes you might need more advanced or customized methods for authentication. PostgreSQL provides several features to support this:
- Authentication Hooks – PostgreSQL allows you to write custom authentication plugins in C to handle authentication requests. This allows you to integrate with custom authentication systems or implement your own authentication logic.
- Certificate Authentication – PostgreSQL supports client certificate authentication, where users authenticate using SSL/TLS certificates rather than passwords. This can be useful for machine-to-machine communication or systems with high security requirements.
- To add an extra layer of security in PostgreSQL, you can use two-factor authentication (2FA). This means combining your password with another factor like a one-time password (OTP) or hardware token. You can do this using external authentication systems like RADIUS or custom authentication plugins.
- Single Sign-On (SSO) – PostgreSQL can integrate with external SSO systems like Kerberos, GSSAPI, or SAML to provide centralized authentication and user management. This allows users to authenticate using their existing corporate or social identities.
Advanced authentication methods need more setup than basic passwords, but offer better security and user experience for specific situations.
Authentication Best Practices
To ensure the security of your PostgreSQL database, follow these authentication best practices:
- Use strong, secure authentication methods like SCRAM-SHA-256. Avoid trust and password methods unless necessary.
- Enable SSL/TLS and require it for all remote connections. Use strong encryption settings and keep your certificates up to date.
- Implement RBAC to apply the principle of least privilege. Only grant users the minimum permissions they need.
- Regularly audit your `pg_hba.conf` and user/role permissions to ensure they align with your security policies.
- Monitor login attempts and database activity for signs of unauthorized access or suspicious behavior.
- Keep your PostgreSQL version and all extensions up to date to get the latest security patches and features.
- Use strong, unique passwords and consider implementing a password policy. Avoid hardcoding passwords in your application code.
- Consider using advanced authentication techniques like 2FA or SSO for high-security environments.
- Educate your users on security best practices, such as choosing strong passwords and not sharing their credentials.
- Have an incident response plan in place to handle potential security breaches or data leaks.
By following these best practices and leveraging PostgreSQL’s authentication and authorization capabilities, you can build a strong security foundation for your database and applications.
Conclusion
PostgreSQL has strong security features to protect your data and manage who can access your database. By learning and correctly setting up these features, you can ensure that only approved users can access your database. Additionally, you can ensure that users can only perform actions that they are authorized to do.
However, authentication is just one aspect of database security. It is important to design your database properly. You should also use secure coding in your apps. Additionally, it is crucial to follow best practices for backup, recovery, and monitoring.
As you grow your PostgreSQL apps, regularly check and update security settings for the right balance of security and usability. Keep up to date on PostgreSQL security features and best practices. Adjust authentication and authorization strategies as needed.
To keep user data safe and protect your business’s reputation, use strong security measures and consistently manage security. This will also help ensure that your apps follow regulations. PostgreSQL provides the tools – it’s up to you to use them effectively.